CPTR 124 Fundamentals of Programming
Teams
In this lab you will construct a prototype for a graphical interface to the game Tic-Tac-Toe.
You are encouraged to work with a partner for this lab. You and your partner should begin thinking about the problems and begin writing the code before lab time.
Background
For a background on the Tic-Tac-Toe game, see http://en.wikipedia.org/wiki/Tic-tac-toe. Your task is to provide a prototype for a graphical interface for Tic-Tac-Toe. This assignment is the first part of a two-part project. In the second part you will implement a game engine that controls the execution of a two-player Tic-Tac-Toe game. This part of the project provides a good start to the look and feel of the overall application, but you will need to adapt your prototype from this assignment to behave properly with your completed game engine.
What to do
For this assignment you must write a Python program that uses Turtle graphics to draw a Tic-Tac-Toe board. Your program must allow a user to click an area of the board to place a player. The possible players are X and O. The figure below shows the application during its execution.
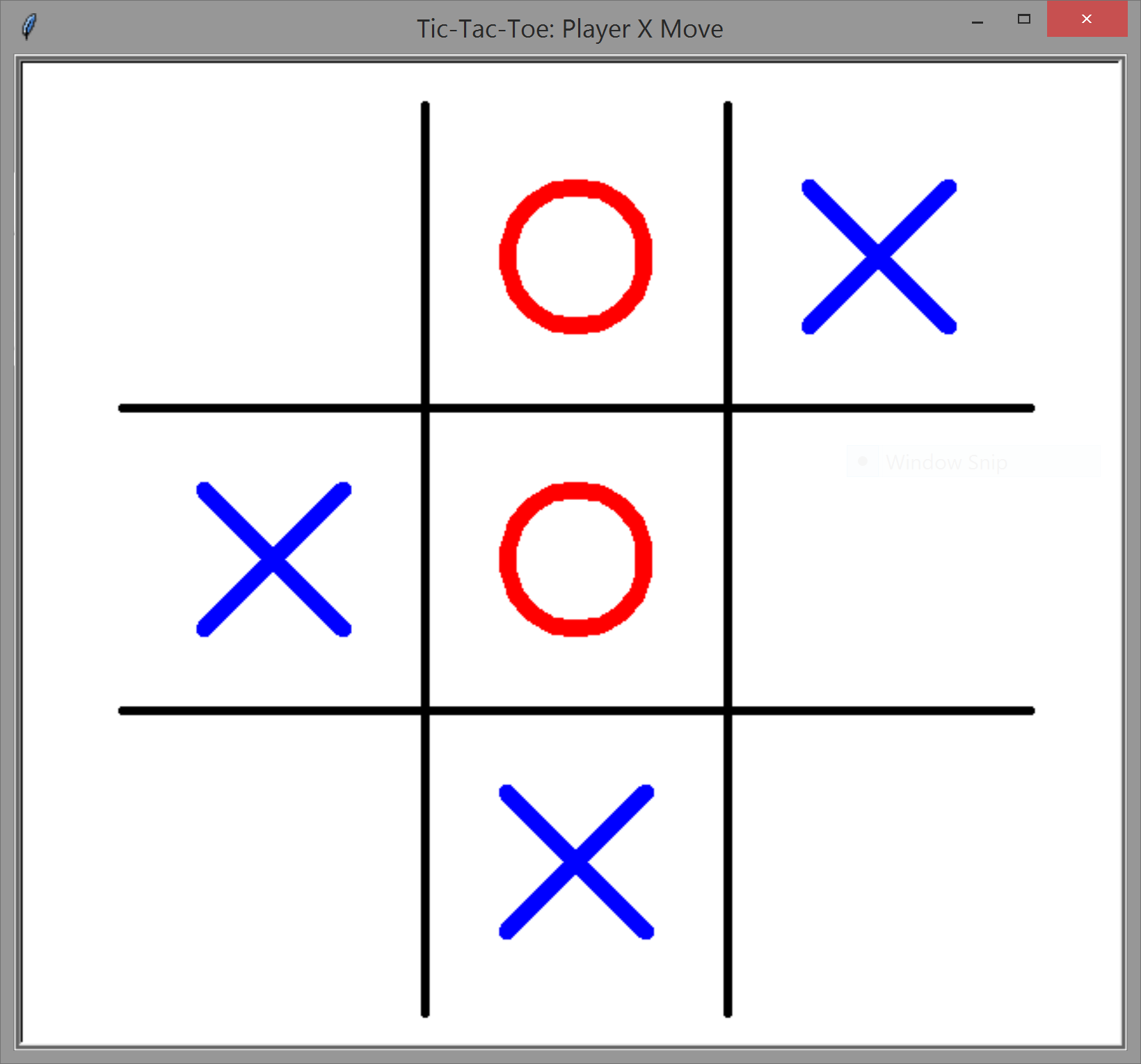
We will identify the areas of the board by the strings shown in the table below.
String |
Meaning |
Role |
|
Top-left square |
Board location |
|
Top-middle square |
Board location |
|
Top-right square |
Board location |
|
Left-middle square |
Board location |
|
Center square |
Board location |
|
Right-middle square |
Board location |
|
Bottom-left square |
Board location |
|
Bottom-middle square |
Board location |
|
Bottom-right square |
Board location |
In this assignment your program will not enforce player alternating turns or prevent placing a mark over an existing mark. Your program will allow the user to select the current player by pressing the X or O key on the keyboard. For this assignment you will not attempt to detect a winning configuration, nor will you attempt to prevent illegal moves. The goal of this assignment is to perfect the visual elements of the game.
You have considerable freedom to write your code as you see fit, but the functions below are highly recommended to get your code up and running and to ease the transition to the second part of the assignment:
-
point_to_square
. This function accepts two parameters,x
andy
, representing the coordinates of a point on the game board. This function returns one of the strings"NorthWest"
,"North"
, etc. that represents a square on the board. In this function you need to provide code that maps a point to a particular square. Your function must return one of the strings exactly as shown above.The goal of this function is to be able to determine which square on the Tic-Tac-Toe game grid corresponds to a particular point in the graphics window. The user can click the mouse at multiple locations within a particular square, and all of those clicks should be treated the same. Your GUI will use this function to determine which square a player chose.
-
square_to_point
. This function accepts one of the strings"NorthWest"
,"North"
, etc. and returns a tuple(x, y)
representing the center of the corresponding square on your game board. This function does the reverse mapping ofpoint_to_square
.This function is useful when when drawing a player's mark on the game board: the lines that make up player X's mark cross at this point, and the circle that makes up player O's mark is centered at this point.
The two functions
point_to_square
andsquare_to_point
work together. When the user clicks anywhere within a valid square on the Tic-Tac-Toe game board, the program can determine which square the user selected viapoint_to_square
. The game itself is concerned only with the nine square positions, not the thousands of possible pixel locations within the graphics window. Presented with a square, the program then can compute exactly where to center a player's mark for drawing. -
drawX
. This function accepts one of the strings"NorthWest"
,"North"
, etc. and draws an X (two big lines that cross) centered within the corresponding square on the game board.This function should use the
square_to_point
function to determine where the Turtle graphics code should draw the centered X mark. -
drawO
. This function accepts one of the strings"NorthWest"
,"North"
, etc. and draws an O (big circle) centered within the corresponding square on the game board.This function should use the
square_to_point
function to determine where the Turtle graphics code should draw the centered O mark.
Your program should run indefinitely until the user closes
the application. This means the last line in your code
should not call done
or
exitonclose
; instead, your last line should
be
This passes control of your program to the Turtle graphics event loop. The event loop monitors users' actions such as clicking the mouse and pressing keys on the keyboard. You have the opportunity to write code that can react to these events. In your program you should write three more functions—the callback functions that you must register with the graphical framework:
-
mouseclick
. This function accepts two parameters that represent the (x, y) components of a point. You can register this function with the graphical framework using the Turtleonscreenclick
function (see the example usingonscreenclick
that we developed in class). This function will need to map the point it receives to its corresponding square and pass this off to eitherdrawX
ordrawO
to actually draw the mark. Yourmouseclick
function must contain logic that determines which player's mark it should draw. -
turnX
. This function establishes that player X has the current move. You can register this function with the graphical framework using the Turtleonkeyrelease
function. YourturnX
function should change the indication of the current player in the window's title bar, and it must change the cursor shape to reflect the current player. This function accepts no parameters. -
turnO
. This function works similarly toturnX
, except it works on player O's behalf.
Notes
- You must use Python's Turtle graphics for all drawing.
- You will find the new (to us) Turtle functions
onscreenclick
,onkeyrelease
,listen
, andgetcanvas
useful. - You should maintain two global variables: one to keep
track of the current player (X or O) and one
to keep track of the window's drawing canvas (see below).
In the next part of the assignment the game engine will
assume the role of keeping track of the current player.
- In order to change the shape of the cursor you must
obtain the current drawing canvas using the
getcanvas
function. The following statement assigns the drawing canvas to a variable namedcanvas
:canvas = getcanvas()Thiscanvas
variable should be a global variable. You then can adjust the cursor's shape as follows:canvas.config(cursor="X_cursor")
makes an X-shaped cursorcanvas.config(cursor="circle")
makes an O-shaped cursor
This is a convenience to the user, providing visual feedback about what mark the next mouse click will produce. In addition, you should indicate the current player in the window's title bar using the
title
function.
Check out
Your finished program will be evaluated for correctness and compliance. Once you have been checked out you may submit your code to eclass.e.southern.edu.