CPTR 124 Fundamentals of Programming
Teams
In this lab you will construct a graphical Tic-Tac-Toe game.
You are encouraged to work with a partner for this lab. You and your partner should begin thinking about the problems and begin writing the code before lab time.
Background
For a background on the Tic-Tac-Toe game, see http://en.wikipedia.org/wiki/Tic-tac-toe. Your task is to write a graphical Tic-Tac-Toe game in C++ using the SGL library's procedural interface. You may begin with blue square graphical program that we developed in class.
What to do
For this assignment you must complete a C++ program that uses the procedural interface to the SGL graphics library to implement a rudimentary Tic-Tac-Toe game. Your program must allow a user to click an area of the board to place a player. The possible players are X and O. The figure below shows the application during its execution.
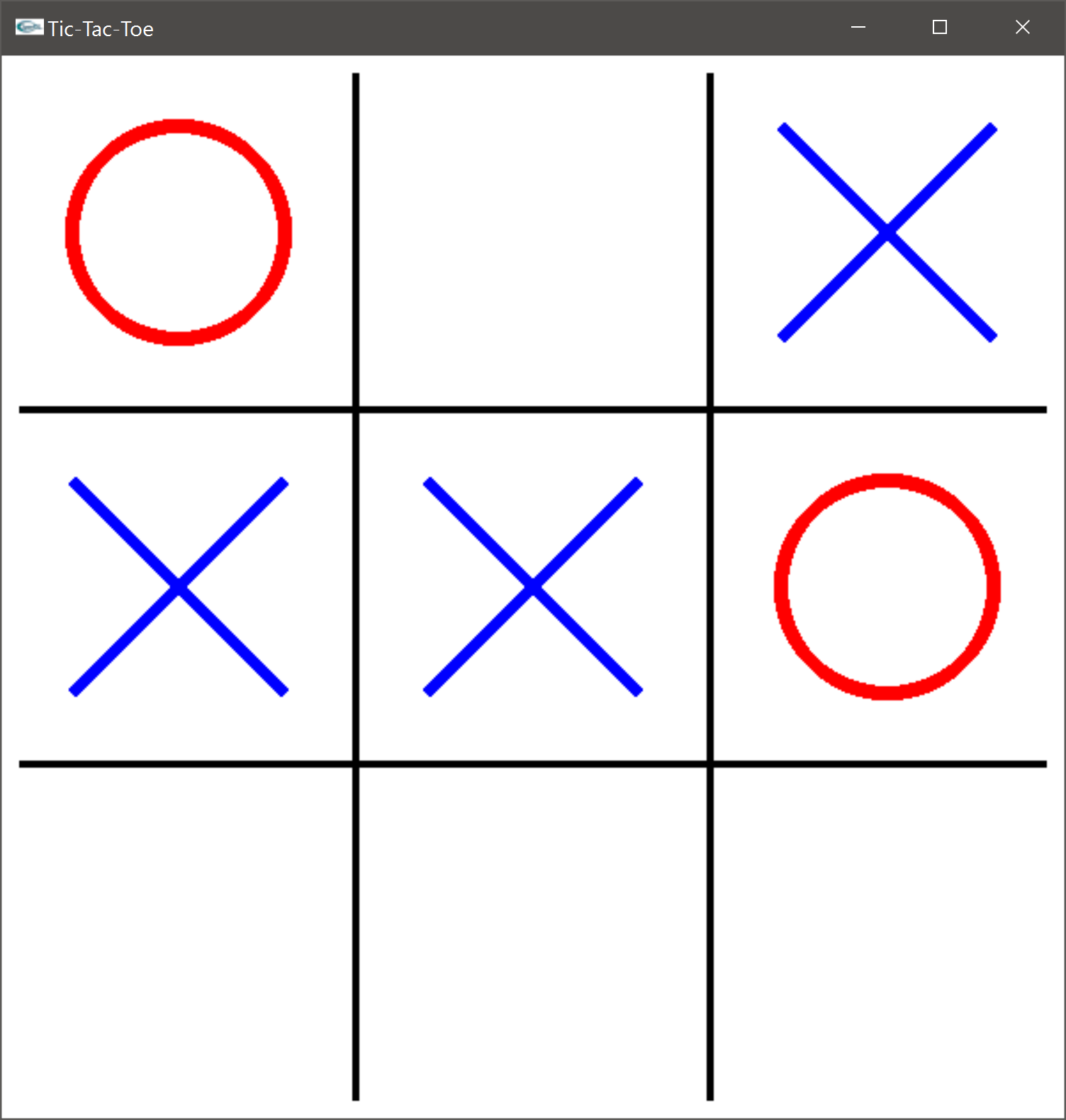
The skeletal code you should begin with is found in tic_tac_toe_skeleton.cpp.
We will identify the areas of the board by the
Square
enumeration types
(Square::Northwest
,
Square::North
, etc.) as shown in the
following table:
Square |
Meaning |
Role |
|
Top-left square |
Board location |
|
Top-middle square |
Board location |
|
Top-right square |
Board location |
|
Left-middle square |
Board location |
|
Center square |
Board location |
|
Right-middle square |
Board location |
|
Bottom-left square |
Board location |
|
Bottom-middle square |
Board location |
|
Bottom-right square |
Board location |
You should use nine global variables to keep track of the
mark corresponding to each of these locations (I called
mine nw
, n
, etc.). Each one of
these variables holds a Player
value. They
all start out with the value Player::None
to indicate at the beginning of the game no square holds
any marks.
You should use a global variable to keep track of whose
turn it is (I called mine current_player
).
This variable holds a Player
value as
well—Player::X
or Player::O
.
In Tic-Tac-Toe X always has the first move.
Your program should enforce player alternating turns and prevent a player from placing a mark over an existing mark.
In this assignment you do not need to check for a winning configuration.
You must complete the functions that appear in the skeletal code.
-
point_to_square
. This function accepts two parameters,x
andy
, representing the coordinates of a point on the game board. This function returns one of theSquare
values (Square::NorthWest
,Square::North
, etc. that represents a square on the board. In this function you need to supply code that maps a point to a particular square. Your function must return aSquare
value.The goal of this function is to be able to determine which square on the Tic-Tac-Toe game grid corresponds to a particular point in the graphics window. The user can click the mouse at multiple locations within a particular square, and all of those clicks should be treated the same. Your GUI will use this function to determine which square a player chose.
-
square_to_point
. This function accepts one of theSquare
valuesSquare::NorthWest
,Square::North
, etc. and assigns to the reference variablesx
andy
the coordinates of the center of the corresponding square on your game board. This function does the reverse mapping ofpoint_to_square
.This function is useful when when drawing a player's mark on the game board: the lines that make up player X's mark cross at this point, and the circle that makes up player O's mark is centered at this point. You can draw an X by drawing two lines with the
sgl::draw_line
function and draw a circle with thesgl::draw_circle
function.The two functions
point_to_square
andsquare_to_point
work together. When the user clicks anywhere within a valid square on the Tic-Tac-Toe game board, the program can determine which square the user selected viapoint_to_square
. The game itself is concerned only with the nine square positions, not the thousands of possible pixel locations within the graphics window. Presented with a square, the program then can compute exactly where to center a player's mark for drawing. -
draw_player
. This function acceptsPlayer
parameter and aSquare
parameter. It draws an X (two lines that cross) or an O (a circle) centered within the corresponding square on the game board. If the player value isPlayer::None
, this function does not draw anything.This function should use the
square_to_point
function to determine where the graphics code should draw the centered mark. -
draw
. This function draws the game grid (four lines) and draws the player marks corresponding to each square usingdraw_player
. -
mouse_pressed
. This function accepts two parameters that represent the (x, y) components of a point. It also accepts asgl::MouseButton
parameter, but your code can ignore it. This function will need to map the point it receives to its corresponding square. If the square is empty (check your global variable keeping track of this square), assign the current player's mark to this square and change the current player to the other player. If the square already has a mark, don't change square's mark and don't change the player.Finally (provided for you in the last line of the skeletal code), this function notifies the graphical framework via
sgl::window_update
to repaint the window so that it draws the new mark on the game board. -
initialize
. This function adjusts all the global variables to their initial state (all squares empty, and current player equal toPlayer::X
). -
main
. You should not touch themain
function.
Check out
Your finished program will be evaluated for correctness and compliance. Once you have been checked out you may submit your code to eclass.e.southern.edu.